Thursday, October 13, 2016
JQuery Form Validation Basics For Blogger Wordpress and All Other Website Platforms
JQuery Form Validation Basics For Blogger Wordpress and All Other Website Platforms
Jquery validate plugin help us to validate user inputs on the client side using class name and attributes. We can use this plugin with Blogger, Wordpress and all other web platforms.
The Jquery validation plugin has a set of predefined rules to validate form fields. Also the plugin allows us to define our own rules to validate specific input fields. In this post I used the predefined rules available in the validate plugin which is enough for a basic contact or registration form.
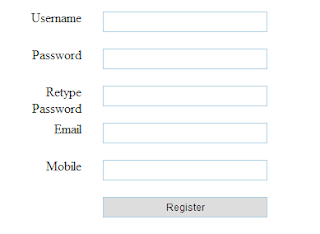
First check out the most using class names and attributes to validate form fields.
1. required : Makes the form field to always required.
2. minlength : Makes the form field to require a given minimum length.
3. maxlength : Makes the form field to limit a given maximum length.
4. min : Makes the form field to require a minimum value.
5. max : Makes the form field to limit a maximum value.
6. email : Makes the form field to require a valid email.
7. digits : Makes the form field to limit integer number only.
8. equalTo : Makes the form field to limit the value as specified fields value.
To Work this validation you have to include two js files in your webpage. Link it either by uploading to your own server or using the provided link.
1. Jquery - Download
1. First Create a HTML form with required fields.
2. Add Some CSS
3. Finally Link the script and call the validate method on form id
The Jquery validation plugin has a set of predefined rules to validate form fields. Also the plugin allows us to define our own rules to validate specific input fields. In this post I used the predefined rules available in the validate plugin which is enough for a basic contact or registration form.
Demo Online
Download Source
Download Source
First check out the most using class names and attributes to validate form fields.
1. required : Makes the form field to always required.
<input type="text" id="username" class="required"/>
2. minlength : Makes the form field to require a given minimum length.
<input type="text" id="username" class="required" minlength="6"/>
3. maxlength : Makes the form field to limit a given maximum length.
<input type="text" id="username" class="required" maxlength="6"/>
4. min : Makes the form field to require a minimum value.
<input type="text" id="username" class="required" min="6"/>
5. max : Makes the form field to limit a maximum value.
<input type="text" id="username" class="required" max="10"/>
6. email : Makes the form field to require a valid email.
<input type="text" id="myemail" class="required email" />
7. digits : Makes the form field to limit integer number only.
<input type="text" id="mobile" class="required digits" />
8. equalTo : Makes the form field to limit the value as specified fields value.
<input type="password" id="pword" class="required" />
<input type="password" id="retypepword" class="required" equalTo="#pword" />
To Work this validation you have to include two js files in your webpage. Link it either by uploading to your own server or using the provided link.
1. Jquery - Download
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script>2. Jquery Validater Plugin - Download
<script src="http://ajax.aspnetcdn.com/ajax/jquery.validate/1.11.0/jquery.validate.js"></script>
Here is an example using Jquery Validate plugin.
1. First Create a HTML form with required fields.
<form id="regform" method="get" action="">
<div class="wrap">
<label id="label" for="username">Username</label>
<input type="text" name="username" id="username" class="required" minlength="6"/>
</div>
<div class="wrap">
<label id="label" for="pass">Password</label>
<input type="password" name="pass" id="pass" class="required" minlength="6"/>
</div>
<div class="wrap">
<label id="label" for="rpass">Retype Password</label>
<input type="password" name="rpass" id="rpass" class="required" equalTo="#pass"/>
</div>
<div class="wrap">
<label id="label" for="useremail">Email</label>
<input type="text" name="useremail" id="useremail" class="required email"/>
</div>
<div class="wrap">
<label id="label" for="mobile">Mobile</label>
<input type="text" name="mobile" id="mobile" class="required digits" minlength="10" maxlength="10"/>
</div>
<div class="wrap">
<label id="label" for="mobile"> </label>
<input type="submit" value="Register"/>
</div>
</form>
2. Add Some CSS
#regform{
margin:0 auto;
width:600px;
padding:14px;
}
input{
font-size:12px;
padding:4px 2px;
border:solid 1px #aacfe4;
width:200px;
margin:2px 5px 10px 10px;
}
#label {
float: left;
width: 100px;
padding: 0 1em;
text-align: right;
}
div{
margin-bottom: .5em;
padding: 0;
display: block;
}
3. Finally Link the script and call the validate method on form id
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js" ></script>
<script src="http://ajax.aspnetcdn.com/ajax/jquery.validate/1.11.0/jquery.validate.js"></script>
<script>
$(function(){
//regform is the form id
$("#regform").validate();
});
</script>
Available link for download